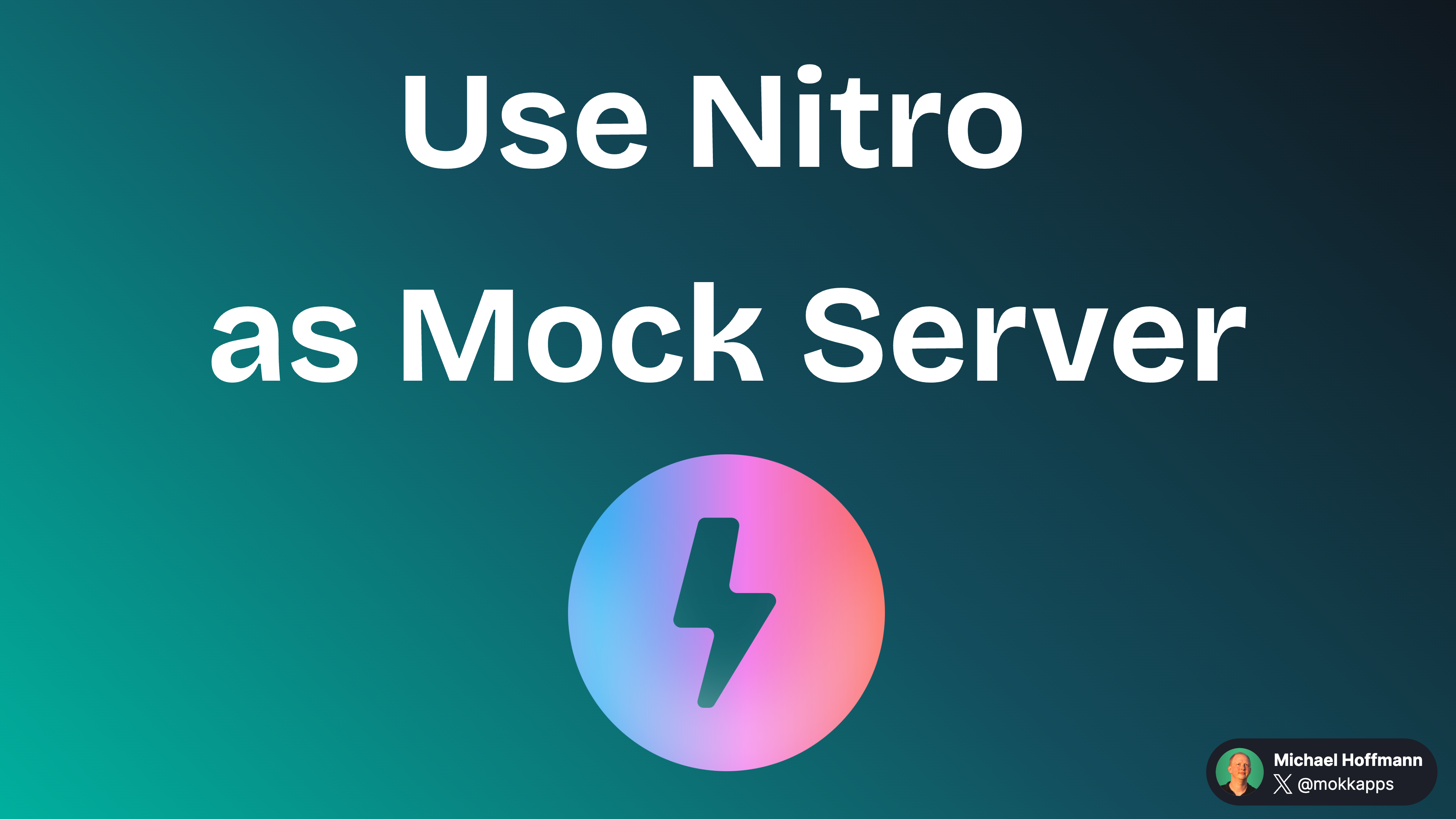
I recently had to implement an end-to-end (E2E) test for a Nuxt.js application that uses Supabase as a backend and authentication provider. The test should log in a user via the Supabase REST API and then test some authenticated pages. In this article, I will show you how to implement this test with Playwright.
The simplest way is to login the user in the E2E test via UI and then test the authenticated pages. But in my case, I wanted to login the user via the REST API to speed up the test execution and/or to avoid flaky tests. I didn't find any content on the internet on how to do this, so I decided to write this article.
Setup File
We use the official docs about authentication as a starting point and define a setup file that logs in the user via the Supabase REST API and stores the session in a file:
The next step is to create a new setup
project in the config and declare it as a dependency for all your testing projects that need authentication:
1export default defineConfig({
2 // ...
3 projects: [
4 { name: 'setup', testMatch: /.*\.setup\.ts/ },
5 {
6 name: 'chromium',
7 use: {
8 ...devices['Desktop Chrome'],
9 },
10 dependencies: ['setup'],
11 },
12 ],
13 // ...
14})
The setup
project will always run and authenticate before all the tests. The session is stored in a file that we can read in the tests for the authenticated pages and set the session in the browser's local storage which is needed by Supabase to authenticate the user:
1import fs from 'fs'
2import { test, expect } from '@playwright/test'
3import { AUTH_FREE_USER_FILE, SUPABASE_APP_ID } from './utils/constants'
4
5test('authenticated page shows logout button', async ({ page, context }) => {
6 const sessionStorage = JSON.parse(fs.readFileSync(AUTH_FREE_USER_FILE, 'utf-8'))
7 await context.addInitScript(
8 (data) => {
9 localStorage.setItem(`sb-${data.appId}-auth-token`, JSON.stringify(data.sessionStorage))
10 },
11 { sessionStorage, appId: SUPABASE_APP_ID }
12 )
13
14 // ... test your authenticated page
15})
If you liked this article, follow me on X to get notified about my new blog posts and more content.
Alternatively (or additionally), you can subscribe to my weekly Vue & Nuxt newsletter:
Use Nitro as Mock Server
Nitro is a sever toolkit that allows you to create web servers with everything you need and deploy them wherever you prefer. It is used in Nuxt 3 to power the server-side part of your Nuxt applications. In this article, I will show you can use Nitro as a mock server for your frontend development.
Simpler Two-Way Binding in Vue With defineModel
The new defineModel compiler macro has available since Vue 3.4 and makes two-way binding much simpler and cleaner.